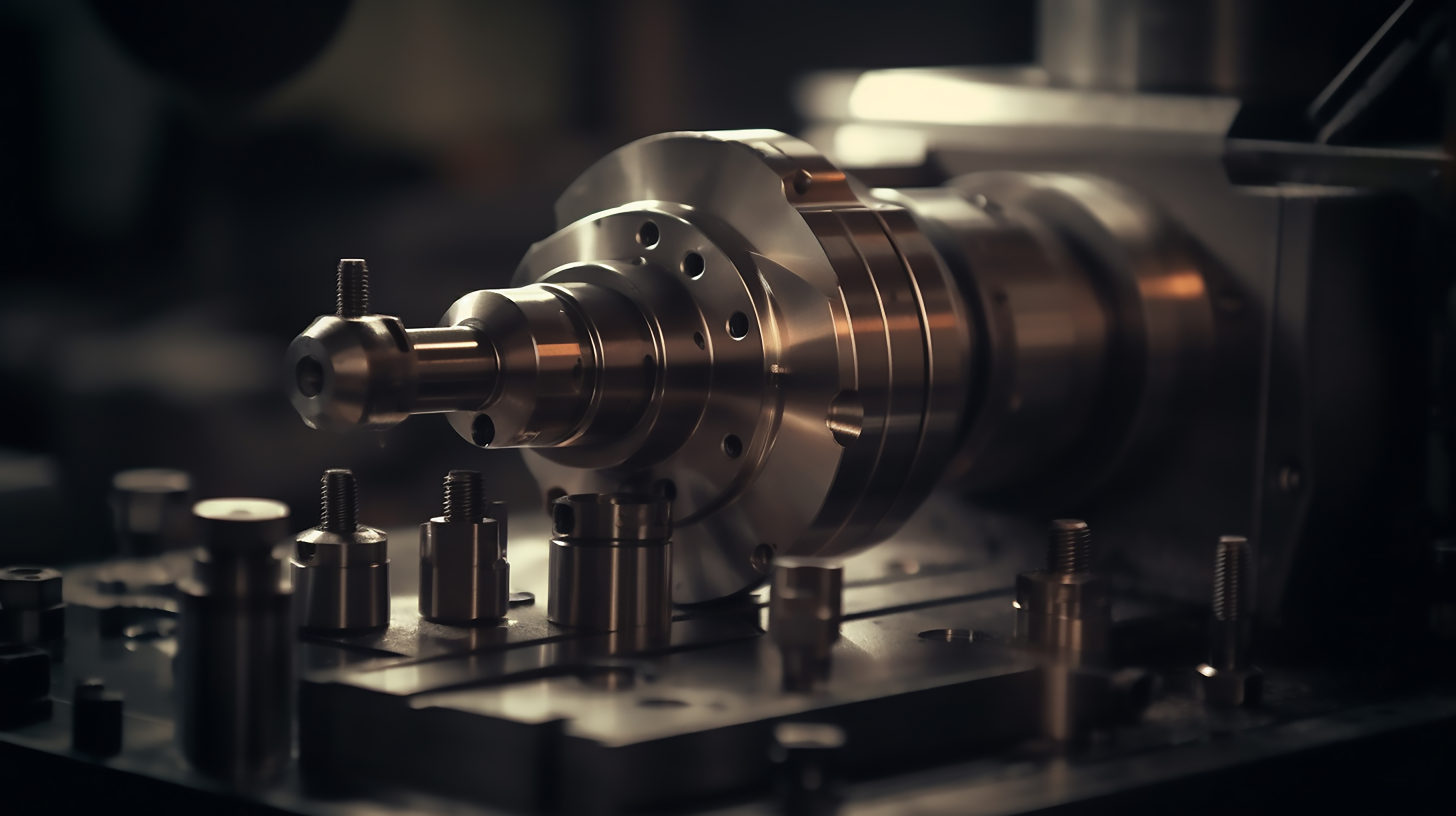
WUXI ANTIRUST TECHNOLOGY Co., Ltd. is an enterprise dedicated to the research and development, production, and sales of rust resistant materials. The company has an experienced and technically proficient R&D team, constantly launching innovative products to meet different market demands. As a professional anti rust material company, Antirust Technology has always been committed to providing customers with high-quality and high-performance anti rust materials to meet the needs of the automotive, heavy machinery, precision machinery, electrical and electronic, material, and military industries.
The company's main products include gas-phase anti rust masterbatch, gas-phase anti rust film, gas-phase anti rust bag, gas-phase anti rust paper, gas-phase anti rust heat shrink film, gas-phase anti rust hollow board, gas-phase anti rust desiccant, gasification water-based anti rust agent, rust remover, anti rust aluminum foil bag, etc. The company adopts advanced production technology and strict quality control system to ensure the quality and stability of its products. The company always adheres to technological innovation as the driving force, customer-oriented, and continuously improves product quality and service level.
In the future, we will continue to increase investment in technology research and development and market expansion, constantly innovate products, and enhance competitiveness. We will adhere to the business philosophy of "integrity cooperation, quality first", provide customers with better rust proof materials and solutions, and contribute to the realization of industrial upgrading and sustainable development.
Our Advantage
-
Equipment
-
Equipment
-
Equipment